Would you like to add/remove columns to a data-table, without the intervention of a developer?
Here we make life easy for the admins !!
Iightning-datatable is used to display the data in the form of a table. We can include/exclude the columns to be displayed in a table using the design:attribute.
Iightning-datatable features the following :
- Displaying and formatting of columns with appropriate data types
- Infinite scrolling of rows
- Inline editing for some data types
- Header-level actions
- Row-level actions
- Resizing of columns
- Selecting of rows
- Sorting of columns by ascending and descending order
- Text wrapping and clipping
- Row numbering column
- Cell content alignment
This blog details the implementation of lightning-datatable, in which the admin can add/remove the columns to be displayed via the lightning record page.
Here we go with an example of displaying list of Accounts in lightning-datatable.
Step 1 :
To edit a page, go to Setup Icon -> Edit Page.
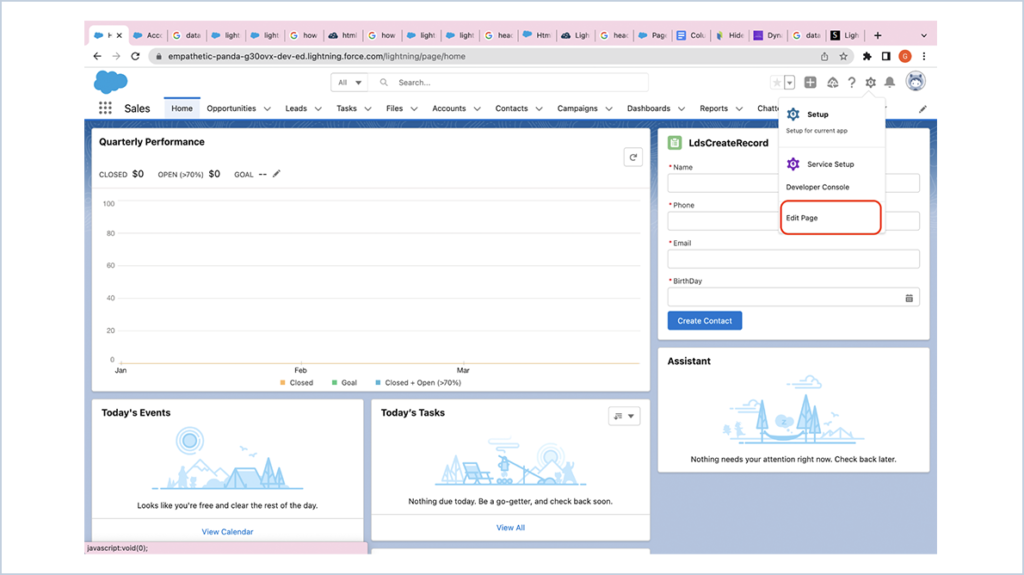
Step 2 :
Drag and Place the custom component on the lightning page.
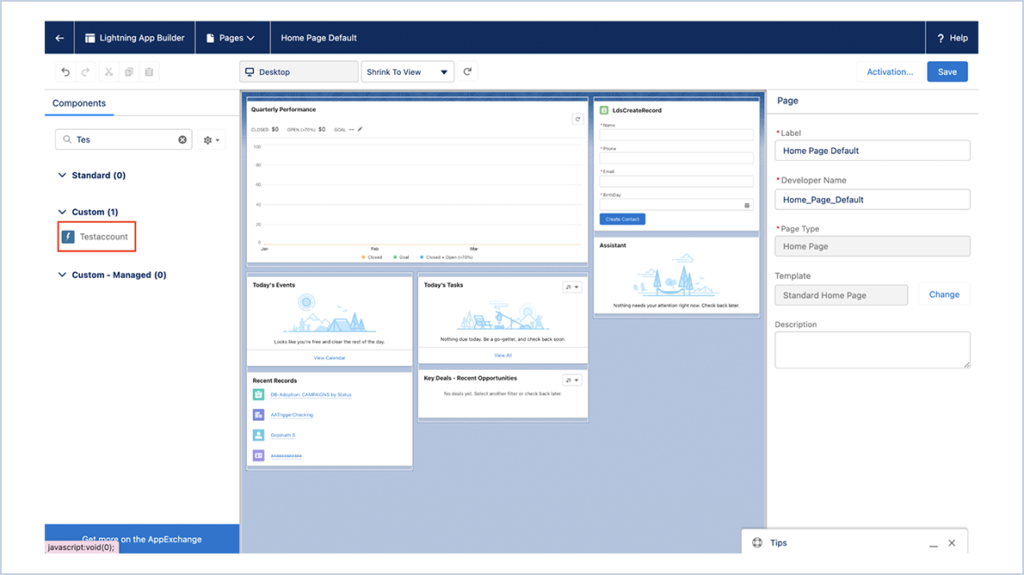
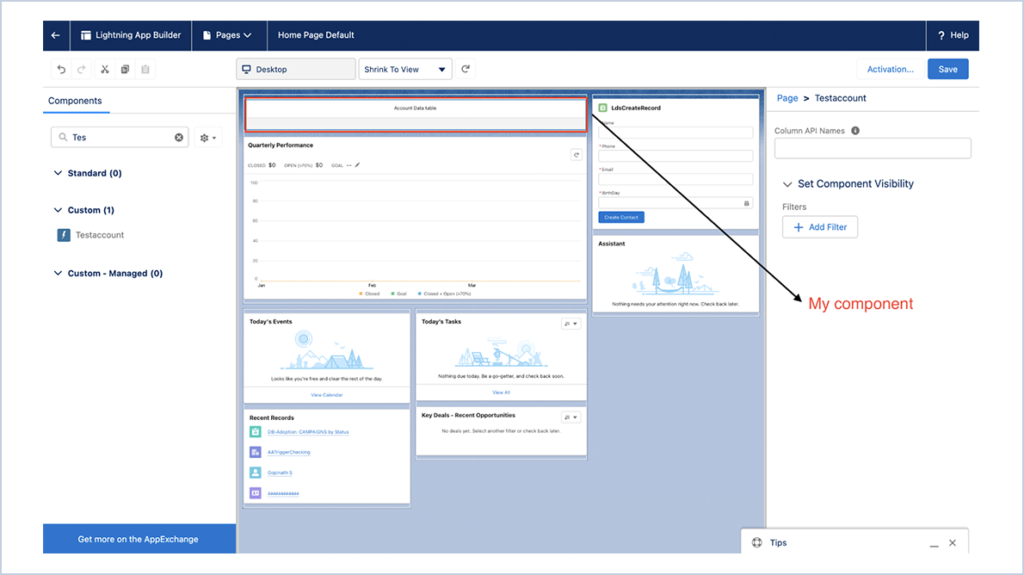
Step 3 :
After placing the component we can see the text box at the top right of the lightning page.
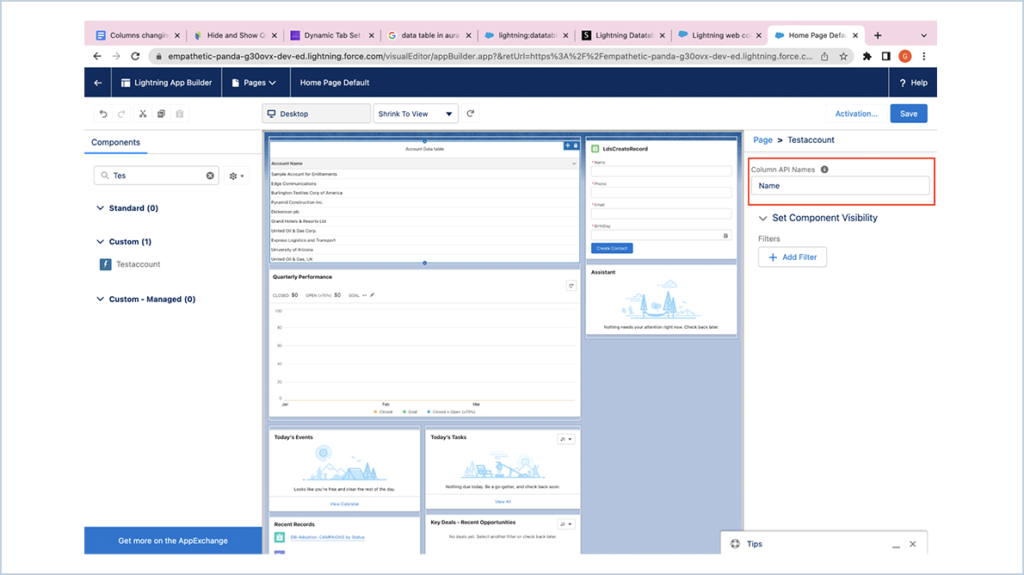
Step 4 :
In the Column API Names textbox enter the field api names of account object in comma separated form. The datatable is constructed by the given field API names in the textbox.
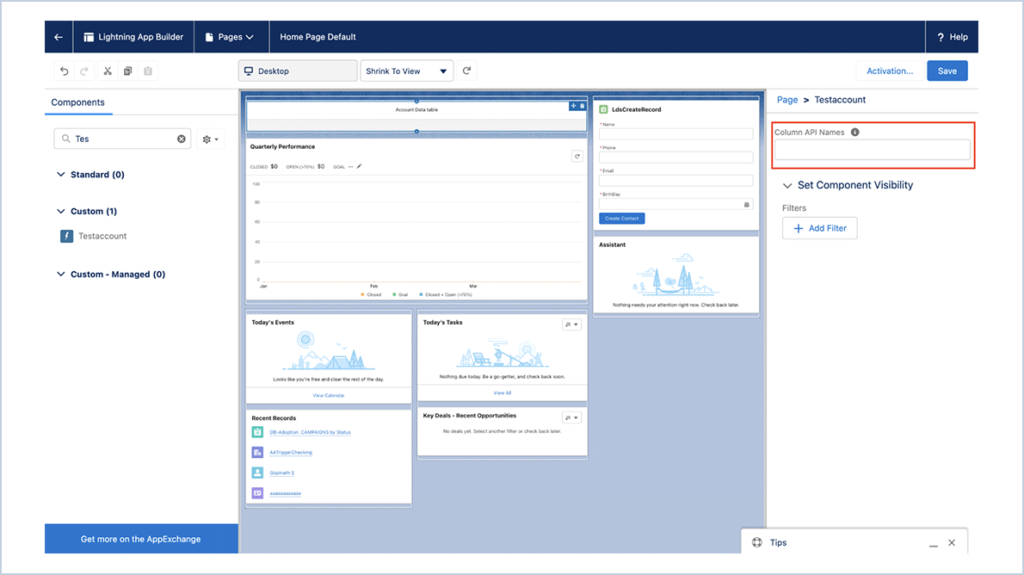
After entering the field api names with comma separated values and click save button at top right.
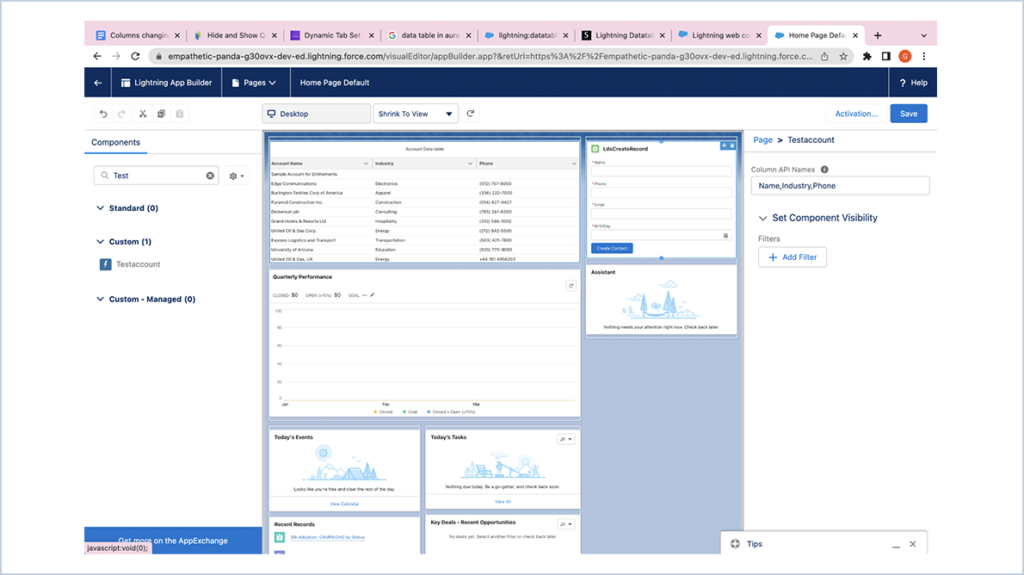
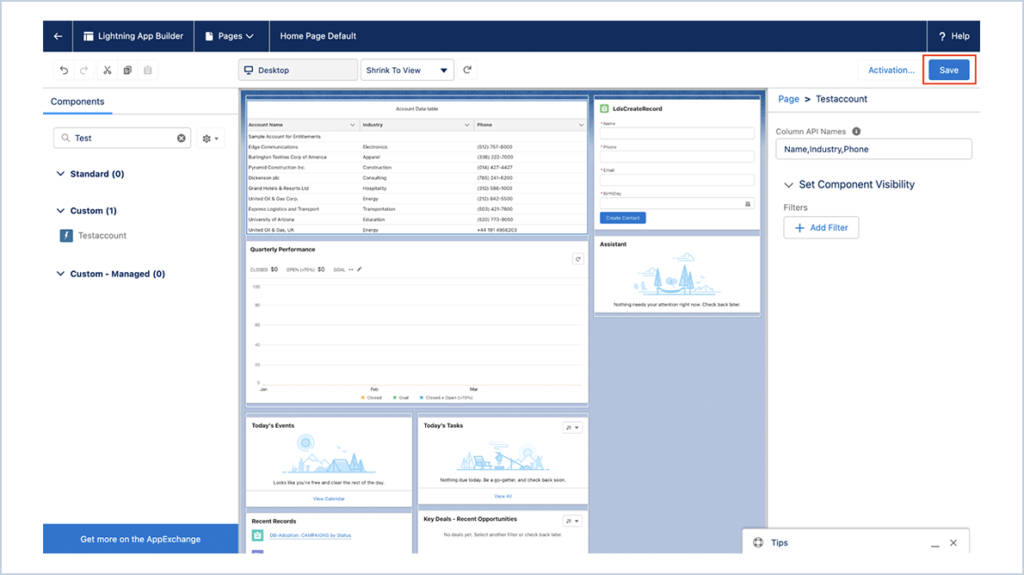
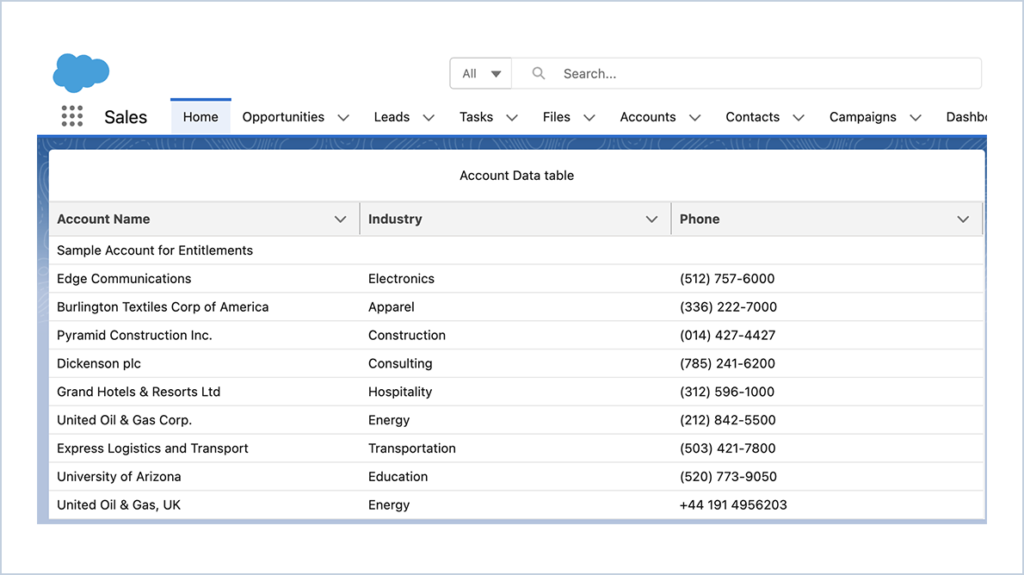
Attached the code to leverage the design:attribute :
Lightning component :
Testaccount.cmp
<aura:component controller="AccountListControllerDesign" implements="force:appHostable,flexipage:availableForAllPageTypes,flexipage:availableForRecordHome,force:hasRecordId,forceCommunity:availableForAllPageTypes,force:lightningQuickAction" access="global">
<aura:attribute name="columnList" type="String" access="public"/>
<aura:attribute type="Account[]" name="acctList"/>
<aura:attribute name="mycolumns" type="List"/>
<header class="slds-modal__header">
<h2>Account Data table</h2>
</header>
<aura:handler name="init" value="{!this}" action="{!c.fetchAcc}"/>
<lightning:datatable data="{! v.acctList }"
columns="{! v.mycolumns }"
keyField="id"
hideCheckboxColumn="true"/>
</aura:component>
Testaccount.design
<design:component >
<design:attribute name="columnList" label="Column API Names" description="Field names of Account object, separated by commas" />
</design:component>
TestaccountController.js
({
fetchAcc : function(component, event, helper) {
helper.fetchAccHelper(component, event, helper);
}
})
TestaccountHelper.js
({
fetchAccHelper : function(component, event, helper) {
var columns = null;
columns = [
{label: 'Account Name', fieldName: 'Name', type: 'text'},
{label: 'Industry', fieldName: 'Industry', type: 'text'},
{label: 'Phone', fieldName: 'Phone', type: 'Phone'},
{label: 'Website', fieldName: 'Website', type: 'url '},
{label: 'AccountNumber', fieldName: 'AccountNumber', type: 'text'},
{label: 'Active', fieldName: 'Active__c', type: 'String'},
{label: 'SLAExpirationDate', fieldName: 'SLAExpirationDate__c', type: 'date'}
];
var selectedColumns = component.get("v.columnList");
if(selectedColumns != undefined) {
// Construct the list of columns from the comma separated string
var listOfColumns = selectedColumns.split(",");
var columnsToDisplay = [];
// Iterate the list of selected columns
for(var i = 0; i < listOfColumns.length; i++) {
for(var j = 0 ; j < columns.length ; j++) {
var fieldNameExisting = JSON.stringify(columns[j].fieldName);
var fieldNameProvided = JSON.stringify(listOfColumns[i]);
if(fieldNameProvided.replaceAll(' ', '') === fieldNameExisting){
columnsToDisplay.push(columns[j]);
}
}
}
}
component.set("v.mycolumns", columnsToDisplay);
var action = component.get("c.fetchAccounts");
action.setParams({
});
action.setCallback(this, function(response){
var state = response.getState();
if (state === "SUCCESS") {
component.set("v.acctList", response.getReturnValue());
}
});
$A.enqueueAction(action);
}
Apex class :
AccountListControllerDesign
public without sharing class AccountListControllerDesign {
@AuraEnabled
public static List <Account> fetchAccounts() {
List<Account> accList = [SELECT Id, Name, AccountNumber, Site, Active__c, BillingState,
Website, Phone, Industry, Type, SLAExpirationDate__c from Account LIMIT 10];
return accList;
}
}
Please be informed that the column names should match the API names, trusting the admins are aware of it.