Use case:
We have an LWC where we display the content dynamically in different columns. We usually use the slds-grid along with slds-size for each column, but the problem arises when the content is dynamic. Sometimes the size may be too small and in some cases, the size may be too large. We need a solution by which the content takes only the required space.
Examples:
1.When the size is large:
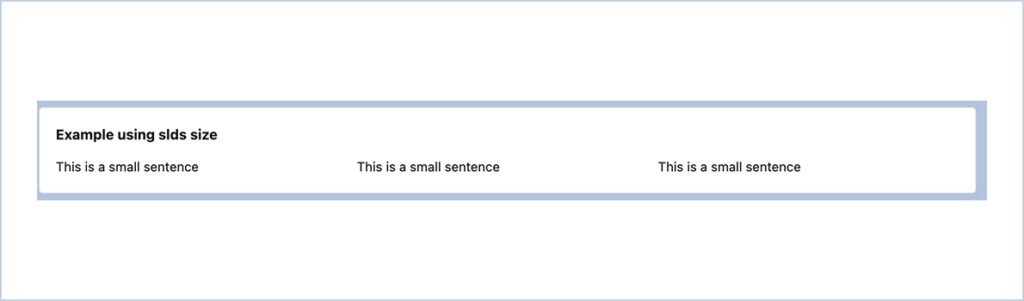
Code:
<template>
<div class="slds-box slds-theme_default">
<div class="slds-text-title_bold slds-p-bottom_small">
Example using slds size
</div>
<div class="slds-grid " title="Sample">
<div class="slds-col slds-size_1-of-3">
<span>This is a small sentence</span>
</div>
<div class="slds-col slds-size_1-of-3">
<span>This is a small sentence</span>
</div>
<div class="slds-col slds-size_1-of-3">
<span>This is a small sentence</span>
</div>
</div>
</div>
</template>
2.When the size is too small:
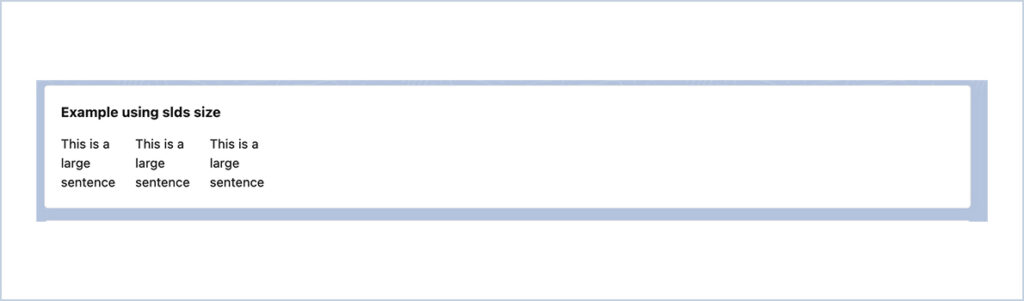
Code:
<template>
<div class="slds-box slds-theme_default">
<div class="slds-text-title_bold slds-p-bottom_small">
Example using slds size
</div>
<div class="slds-grid " title="Sample">
<div class="slds-col slds-size_1-of-12">
<span>This is a large sentence</span>
</div>
<div class="slds-col slds-size_1-of-12">
<span>This is a large sentence</span>
</div>
<div class="slds-col slds-size_1-of-12">
<span>This is a large sentence</span>
</div>
</div>
</div>
</template>
Solution:
The alternative we have for this is to use slds-grow-none along with the column instead of slds-size. By using slds-grow-none, we let the column shrink to the width of the content inside of it.
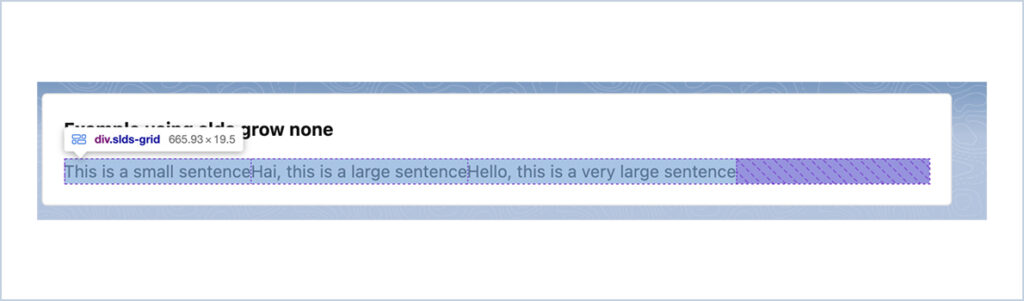
Now we can see that the contents takes only the required space
Code:
<template>
<div class="slds-box slds-theme_default">
<div class="slds-text-title_bold slds-p-bottom_small">
Example using slds grow none
</div>
<div class="slds-grid " title="Sample">
<div class="slds-col slds-grow-none">
<span>This is a small sentence</span>
</div>
<div class="slds-col slds-grow-none ">
<span>Hai, this is a large sentence</span>
</div>
<div class="slds-col slds-grow-none ">
<span>Hello, this is a very large sentence</span>
</div>
</div>
</div>
</template>
But yes, it looks a bit clumsy. Here comes our saviour gutters. Instead of giving separate padding for each column we could just use slds-gutters which would give equal space between columns that helps separate content.
Snapshots:
Desktop:
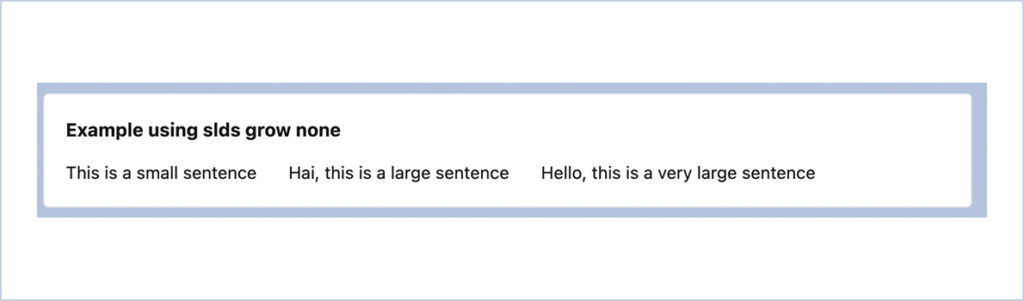
Mobile:
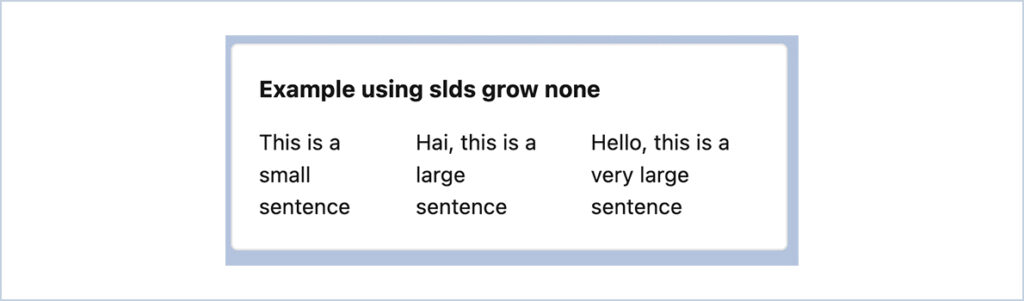
Code:
<template>
<div class="slds-box slds-theme_default">
<div class="slds-text-title_bold slds-p-bottom_small">
Example using slds grow none
</div>
<div class="slds-grid slds-gutters_small" title="Sample">
<div class="slds-col slds-grow-none">
<span>This is a small sentence</span>
</div>
<div class="slds-col slds-grow-none ">
<span>Hai, this is a large sentence</span>
</div>
<div class="slds-col slds-grow-none ">
<span>Hello, this is a very large sentence</span>
</div>
</div>
</div>
</template>