Field Set :
A field set is a grouping of fields for an object. Field sets are supported for all standard objects and custom object fields.
Field sets can be added to a Visualforce page or LWC and can be used to query and display the records. Administrators can change the fields displayed on a page by adding or removing or rearranging the fields in a field set without changing any code.
Configuring a Field Set :
Step 1: Go to Setup -> Object Manager
Step 2: Select the Object for which a Field Set to be created
Step 3: Select Field Set on the left column
Step 4: Click on New give appropriate Field Set Label, Name and Where is this used?
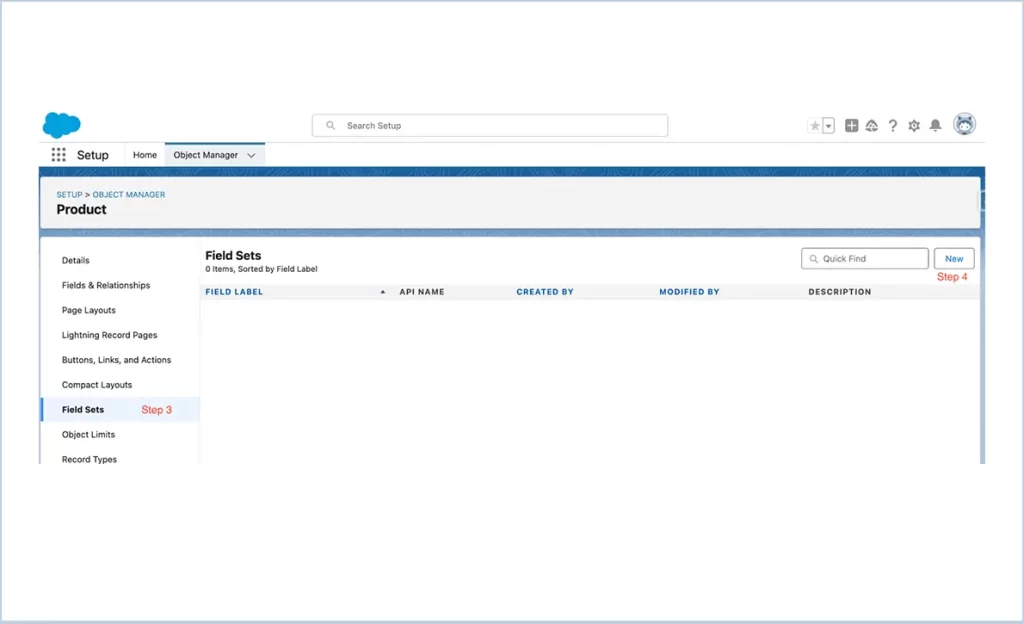
Field Set Label : The name presented to subscribers who install the field through a managed package.
Field Set Name : Used by developers to reference the field set in code.
Where is this used : Provides a brief description of where the Field Set is used and for what purpose.
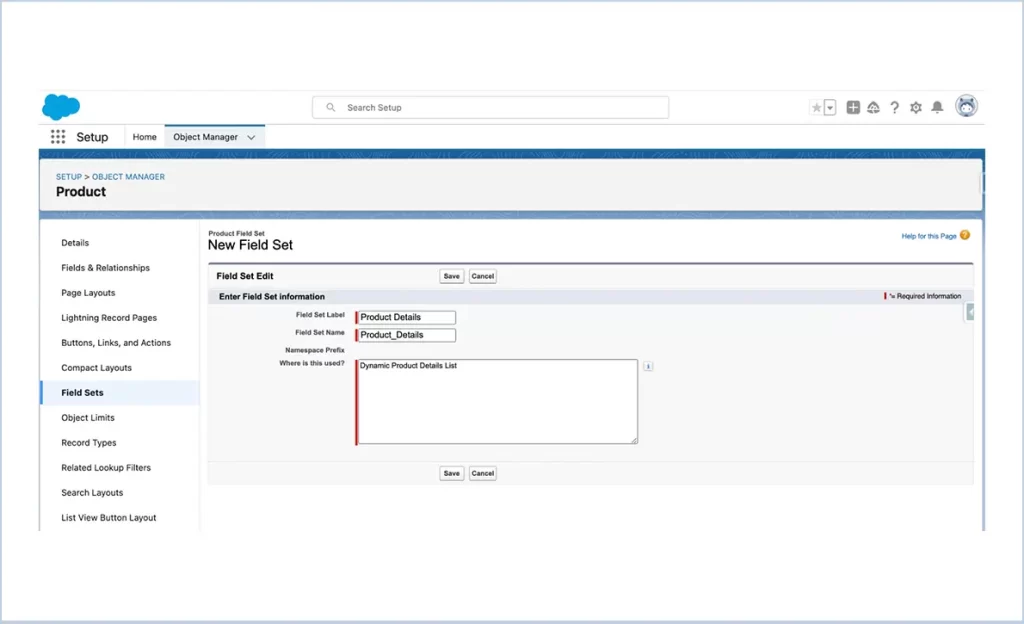
Step 5: Click the Save Button and it will Navigate to the field set build page.
Step 6: Drag and Drop the required fields to field set drop box
On each fields on field set box right corner you can find the 🔧from which we can define if the field is required for the field set ( Defined by * symbol)
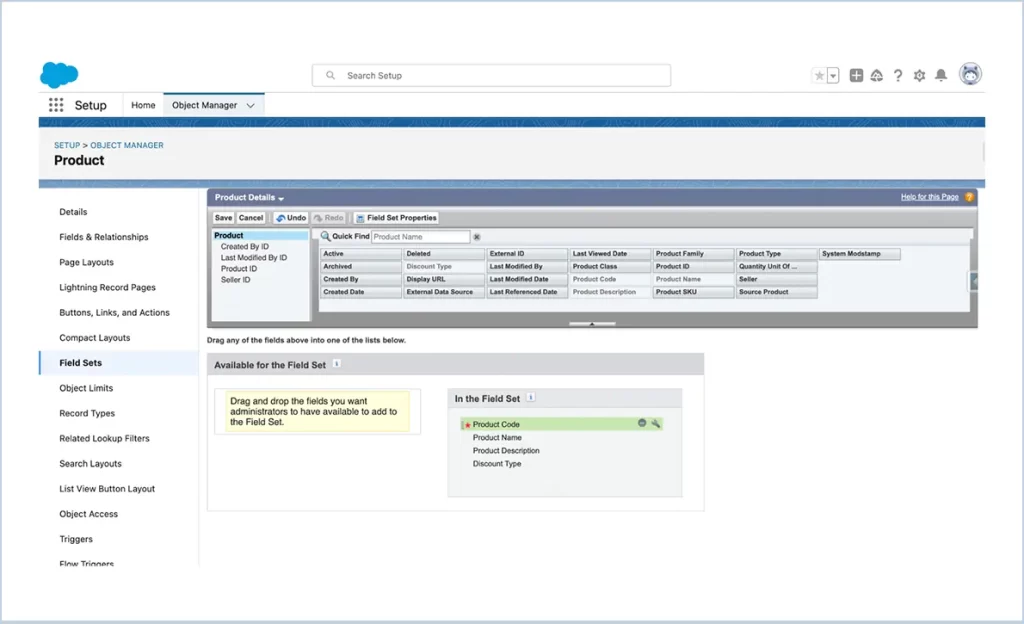
FieldSetMember Class in Apex :
Salesforce provided in-built methods to access the fields in a Field Set in apex classes.
Schema.FieldSetMember class provides the details about fields contained within a field set, such as the field label, type, a dynamic SOQL-ready field path, and so on. The following code shows how to get a collection of field set member describe result objects for a specific field set on an sObject
List<Schema.FieldSetMember> fields = Schema.SObjectType.Account.fieldSets.getMap().get('field_set_name').getFields();
The above code returns the fields present in the field set.
For more information about the FieldSetMember class click
Creating a dynamic query by using field sets
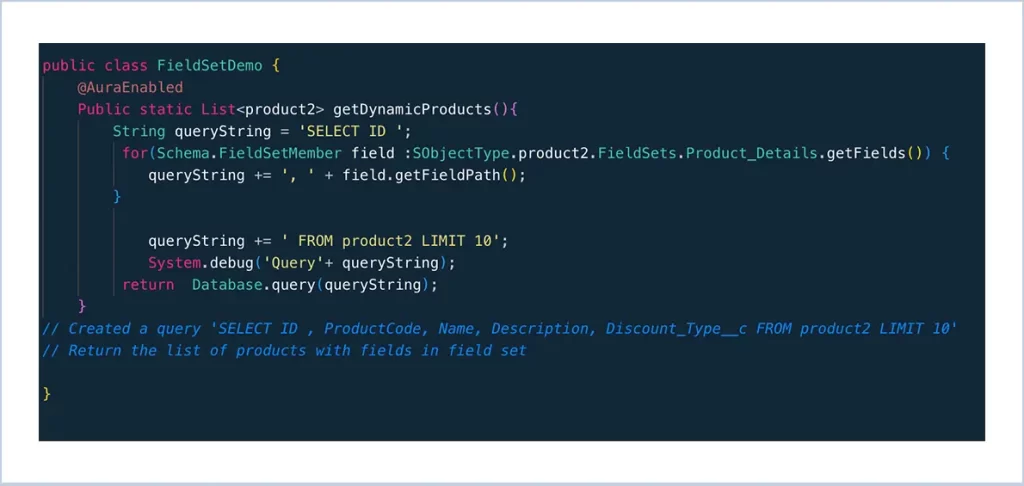
In our scenario Product Detail field sets contains Product Code, Product Name, Product Description & Discount Type fields
Field Set in LWC :
Creating a Data Table by Using Field Sets in LWC
1. fieldSet.html
<template>
<lightning-card title={title}>
<!-- Data Table Using Field Sets
Data = Account Records
Columns = Fields from Field Sets
-->
<lightning-datatable key-field="id" data={data} columns={columns}>
</lightning-datatable>
</lightning-card>
</template>
2.dataTableUsingFieldSets.js
import { LightningElement,wire} from 'lwc';
import fieldSetClass from '@salesforce/apex/DataTableUsingFieldSets.getFieldLableAndFieldAPI';
import getProductRecords from '@salesforce/apex/DataTableUsingFieldSets.getProductRecords';
export default class DataTableUsingFieldSets extends LightningElement {
columns = [];
title = 'Field Sets on Lightning Table';
data = [];
connectedCallback() {
this.createDataTable();
}
//Creating a dynamic table
createDataTable() {
fieldSetClass().then((data) => {
// Collection of fields in Field Sets
let fieldSet = JSON.parse(data);
let column = [];
// Iterating over each fields in field sets
for (var i = 0; i < fieldSet.length; i++) {
// Constructing a array map with field Label and API Name
column.push({
label: Object.keys(fieldSet[i])[0],
fieldName: Object.values(fieldSet[i])[0]
});
}
this.columns = column;
console.log(JSON.stringify(this.columns))
})
}
//Get Records and Bind in array
@wire(getProductRecords)
getProductRecords({
data,
error
}) {
if (data) {
this.data = data;
} else if (error) {
alert('Unable to Fetch the Records');
}
}
}
3. DataTableUsingFieldSets.apxc
public class DataTableUsingFieldSets {
// Method to Create a Data Table Using Fields in Field Sets
@AuraEnabled(cacheable=true)
public static string getFieldLableAndFieldAPI(){
// Creating a List of Map that contain fields Label and API Name
List<Map<String ,String>> fieldsLabelApiName = new List<Map<String ,String>>();
// Iterating Over the Credential Field Sets to take each fields in Field sets
for(Schema.FieldSetMember fieldset : SObjectType.product2.FieldSets.Product_Details.getFields()) {
// Map to store each field Label and API Name
Map<String ,String> lableAPIName = new Map<String ,String>();
lableAPIName.put(fieldset.getLabel(),fieldset.getFieldPath());
fieldsLabelApiName.add(lableAPIName);
}
//Return as to JS
return JSON.serialize(fieldsLabelApiName);
}
// Method to Collect Account Record using Field Sets
@AuraEnabled(cacheable=true)
public static List<product2> getProductRecords() {
try {
String queryString = 'SELECT id';
for (Schema.FieldSetMember fieldSet : SObjectType.product2.FieldSets.Product_Details.getFields()) {
queryString += ',' + fieldSet.getFieldPath();
}
queryString += ' from product2 order by Createddate desc limit 10';
return Database.query(queryString);
} catch (Exception e) {
throw new AuraHandledException(e.getMessage());
}
}
}
Output :
Data Table using the fields in Field Sets
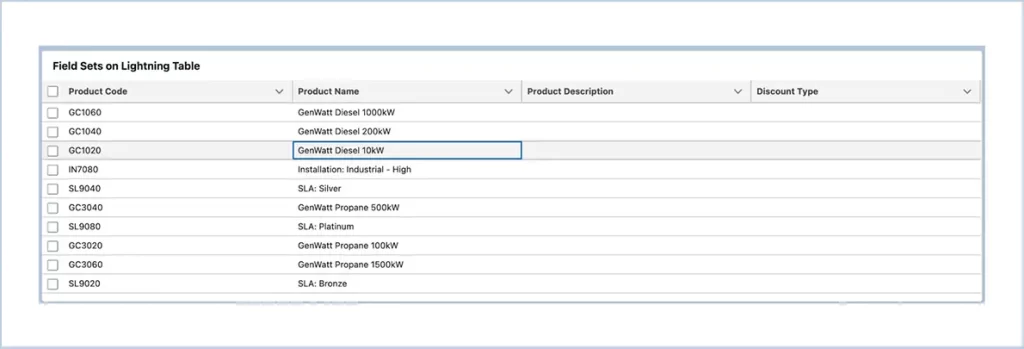
Advantages :
- We can dynamically add or remove fields from Field Sets without modifying the code changes
- We can have additional feature like making a field required for particular Field Sets depending upon the use case (For example if we have a form, instead of marking the field required at the form level, it can be done the Field Set itself)
- Query larger number of fields can be made simple by adding the requested field in Field Sets and just calling Field Sets instead of huge query
Limitations :
1. Limited to One Level of Parent Fields
Field Sets currently support only one level of parent fields, while SOQL allows for up to five parent levels. It would be beneficial to have similar flexibility with field sets. As a workaround, you can create one or more formula fields on the object or its parent to retrieve the additional information and then include those formula fields in the field set.
2. Limitations of Field Sets in Out-of-the-Box Features
Field sets cannot be utilised in out-of-the-box features like Flow. This limitation means you cannot dynamically pull in Field Sets directly within Flow designs, which restricts the flexibility that field sets provide in other areas of Salesforce. As a result, you’ll have to manually configure fields in your Flow, limiting the ease of maintenance and consistency that field sets typically offer.