In this blog, I’ll go over a very interesting feature that lwc offers.
I’ll show you how to make a dependent picklist in lwc without using apex. LWC provides the getPicklistValuesByRecordType module, which retrieves the picklist values from the object for a specific RecordType.
This allows for the retrieval of multiple values based on the controlling field. We can obtain the same information as we do via the record-edit-form in lwc.
We can retrieve picklist values and dependent values associated with a record type using the lightning/uiObjectInfoApi.
It requires the parameters objectApiName and RecordTypeId.
import { getPicklistValuesByRecordType } from ‘lightning/uiObjectInfoApi’;
To retrieve picklist values, we do not need to make an apex call or use schema functions.Additionally, there are some limitations to obtaining dependent values via Apex’s metadata API, such as Each user in salesforce has a limited ability to access the metadata API. Due to this limitation, the metadata API cannot be used to retrieve picklist values on large systems.
LWC Component to Display Dependant PickList
CarDetails.lwc
<template>
<div class=" slds-card">
<div class="slds-grid slds-gutters slds-p-top_small slds-p-bottom_large slds-wrap">
<div class="slds-col slds-size_4-of-12">
</div>
<div class="slds-col slds-size_4-of-12">
<lightning-combobox name="Car" label="Car" placeholder="Select Car Name" options={carValues} required
onchange={handleCarChange} value={selectedCarValue}>
</lightning-combobox>
</div>
<div class="slds-col slds-size_4-of-12">
</div>
<div class="slds-col slds-size_4-of-12">
</div>
<div class="slds-col slds-size_4-of-12">
<lightning-combobox name="Car Color" label="Car Color" placeholder="Select Car Color" options={carColorValues} required
onchange={handleCarColorChange} value={selectedCarColorValue}>
</lightning-combobox>
</div>
<div class="slds-col slds-size_4-of-12">
</div>
</div>
</div>
</template>
CarDetails.js
import { LightningElement, wire } from 'lwc';
import { getPicklistValuesByRecordType } from 'lightning/uiObjectInfoApi';
export default class CarDetails extends LightningElement {
carValues;
carColorValues;
selectedCarValue = '';
picklistValuesObj;
selectedCarColorValue = '';
// method to get Picklist values based on record type and dependant values too
@wire(getPicklistValuesByRecordType, { objectApiName: 'Car__c', recordTypeId: '0125g0000003W2vAAE' })
newPicklistValues({ error, data }) {
if (data) {
this.error = null;
this.picklistValuesObj = data.picklistFieldValues;
console.log('data returned' + JSON.stringify(data.picklistFieldValues));
let carValueslist = data.picklistFieldValues.Car_Name__c.values;
let carValues = [];
//Iterate the picklist values for the car name field
for (let i = 0; i < carValueslist.length; i++) {
carValues.push({
label: carValueslist[i].label,
value: carValueslist[i].value
});
}
this.carValues = carValues;
console.log('data car values' + JSON.stringify(this.carValues));
}
else if (error) {
this.error = JSON.stringify(error);
console.log(JSON.stringify(error));
}
}
handleCarChange(event) {
this.selectedCarValue = event.detail.value;
if (this.selectedCarValue) {
let data = this.picklistValuesObj;
let totalCarColorValues = data.Car_Color__c;
//Getting the index of the controlling value as the single value can be dependant on multiple controller value
let controllerValueIndex = totalCarColorValues.controllerValues[this.selectedCarValue];
let colorPicklistValues = data.Car_Color__c.values;
let carColorPicklists = [];
//Iterate the picklist values for the car name field
colorPicklistValues.forEach(key => {
for (let i = 0; i < key.validFor.length; i++) {
if (controllerValueIndex == key.validFor[i]) {
carColorPicklists.push({
label: key.label,
value: key.value
});
}
}
})
console.log(' data carColorPicklists' + JSON.stringify(carColorPicklists));
if (carColorPicklists && carColorPicklists.length > 0) {
this.carColorValues = carColorPicklists;
}
}
}
handleCarColorChange(event){
this.selectedCarColorValue = event.detail.value;
}
}
Image 1 :
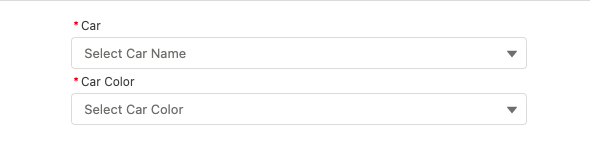
Image 2:
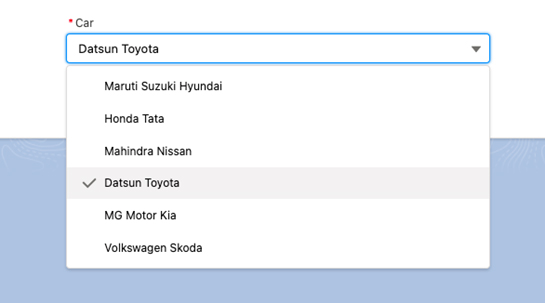
Image 3:
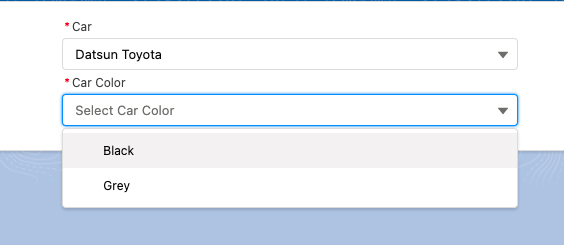
Image 4:
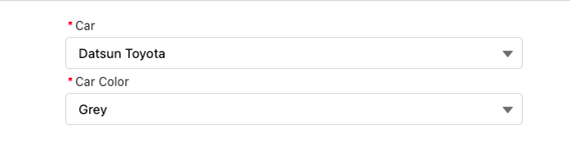
Image 5:
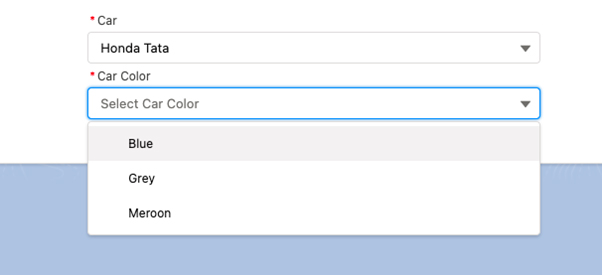
lightning/uiObjectInfoApi – Pickist JSON Response
{
"Car_Color__c": {
"controllerValues": {
"Maruti Suzuki Hyundai": 0,
"Honda Tata": 1,
"Mahindra Nissan": 2,
"Datsun Toyota": 3,
"MG Motor Kia": 4,
"Volkswagen Skoda": 5
},
"defaultValue": null,
"url": "/services/data/v52.0/ui-api/object-info/Car__c/picklist-values/0125g0000003W2vAAE/Car_Color__c",
"values": [
{
"attributes": null,
"label": "White",
"validFor": [
0,
2,
4
],
"value": "White"
},
{
"attributes": null,
"label": "Black",
"validFor": [
0,
3,
4
],
"value": "Black"
},
{
"attributes": null,
"label": "Blue",
"validFor": [
0,
1,
2,
4
],
"value": "Blue"
},
{
"attributes": null,
"label": "Grey",
"validFor": [
1,
3,
4
],
"value": "Grey"
},
{
"attributes": null,
"label": "Meroon",
"validFor": [
1,
2,
4
],
"value": "Meroon"
}
]
},
"Car_Name__c": {
"controllerValues": {},
"defaultValue": null,
"url": "/services/data/v52.0/ui-api/object-info/Car__c/picklist-values/0125g0000003W2vAAE/Car_Name__c",
"values": [
{
"attributes": null,
"label": "Maruti Suzuki Hyundai",
"validFor": [],
"value": "Maruti Suzuki Hyundai"
},
{
"attributes": null,
"label": "Honda Tata",
"validFor": [],
"value": "Honda Tata"
},
{
"attributes": null,
"label": "Mahindra Nissan",
"validFor": [],
"value": "Mahindra Nissan"
},
{
"attributes": null,
"label": "Datsun Toyota",
"validFor": [],
"value": "Datsun Toyota"
},
{
"attributes": null,
"label": "MG Motor Kia",
"validFor": [],
"value": "MG Motor Kia"
},
{
"attributes": null,
"label": "Volkswagen Skoda",
"validFor": [],
"value": "Volkswagen Skoda"
}
]
}
}
Salesforce Implementation | LWC | Dependent PickList | innovalleyworks